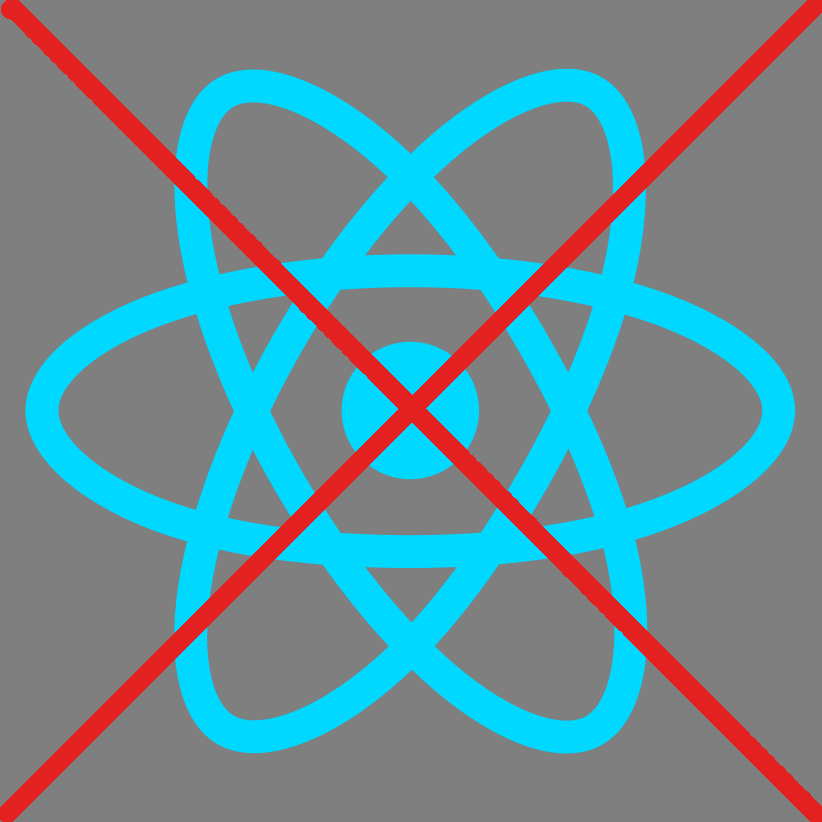
Notes
- This is not a talk on React - the web framework put out by facebook
*
Asynchronous javascript:
function asyncTask(args, callback) {
// do stuff
callback()
}
asyncTask
asyncTask(args, function() {
// task is done here!
})
asyncTask1(args, function() {
asyncTask2(args, function() {
asyncTask1(args, function() {
// task is done here!
})
})
})
var result1=false; result2=false; // state!!
asyncTask1(function(){a1=true; doAction()}
asyncTask2(function(){a2=true; doAction()}
function doAction() {
if (a1 && a2) {
…
}
}
asyncTask1.then(asyncTask2).then(function() {
// success
}, function(err) {
// error
})
Promise.all(asyncTask1, asyncTask2).then(...)
From: http://www.slideshare.net/stefanmayer13/functional-reactive-programming-with-rxjs
Think of an observable as a collection-in-time
forEach
filter
map
Iterating over an array
for (var i=0; i < a.length; i++) {
item = a[i];
// item.doAction()
}
a.forEach(function(item) {
// item.doAction()
})
See the Pen Collection | Iden by Brian Leathem (@bleathem) on CodePen.
See the Pen Observable by Brian Leathem (@bleathem) on CodePen.
Reactive Extensions for JavaScript
…is a set of libraries to compose asynchronous and event-based programs using observable collections and Array#extras style composition in JavaScript
map
reduce
mergeAll
reduce
zip
map
.map(function(x) {
return {
id: x.id
, color: 'green'
, size: x.size
, type: 'square'
};
});
Map each shape
into a green square
of the same size
map
See the Pen Operating on a Collection by Brian Leathem (@bleathem) on CodePen.
map
See the Pen Map an Observable by Brian Leathem (@bleathem) on CodePen.
mergeAll
.map(function(x) {
var y = _.clone(x);
y.id = y.id + 80;
y.color = 'green';
var z = _.clone(x);
y.size = y.size / 1.5;
z.size = z.size / 1.5;
return [y, z];
})
.mergeAll();
See the Pen Map a nested Collection by Brian Leathem (@bleathem) on CodePen.
mergeAll
See the Pen MergeAll a Collection by Brian Leathem (@bleathem) on CodePen.
mergeAll
See the Pen MergeAll an Observable by Brian Leathem (@bleathem) on CodePen.
flatMap
flatMap
is a shorthand for a map
followed by a mergeAll
.
.flatMap(function(x) {
var y = _.clone(x);
y.id = y.id + 80;
y.color = 'green';
var z = _.clone(x);
y.size = y.size / 1.5;
z.size = z.size / 1.5;
return [y, z];
});
reduce
var outputData = inputData
.reduce(function(acc, x) {
return {
id: x.id
, color: 'green'
, size: acc.size + x.size
, type: 'square'
};
}, {size: 0});
reduce
See the Pen Reduce a Collection by Brian Leathem (@bleathem) on CodePen.
reduce
See the Pen Reduce an Observable by Brian Leathem (@bleathem) on CodePen.
zip
var outputData = Rx.Observable.zip(
input1Data,
input2Data,
function(x1, x2) {
return {
id: x1.id
, color: x1.color
, size: x2.size
, type: x2.type
};
});
zip
See the Pen Zip an Observable by Brian Leathem (@bleathem) on CodePen.
Observable in JavaScript proposal presented to TC-39 (JS standards committee) today. Advanced to Stage 1 (Proposal). https://t.co/sBuazdM7vR
— Jafar Husain (@jhusain) May 29, 2015
var source = Rx.Observable.create(function (observer) {
observer.onNext(42);
observer.onCompleted();
// Optional: only return this if cleanup is required
return function () {
console.log('disposed');
};
});
Rx.Observable.create(function(observer) {
var element = document.getElementById("box1");
element.addEventListener("mousemove", function(event) {
observer.onNext(event);
}, false);
});
fromEvent
helpervar element = document.getElementById("box1");
Rx.Observable.fromEvent(element, 'mousemove');
Rx.Observable.fromEvent(element, 'mousemove')
.subscribe(
function(event) {
console.log(event);
},
function(error) {
console.log(error);
},
function() {
// stream completed
}
http://reactive-extensions.github.io/learnrx/
Rx.Observable.fromEvent(element, 'mousemove')
.filter(function(event) {
return event.target.classList.contains('myClass');
})
.subscribe(...);
See the Pen Event Listener by Brian Leathem (@bleathem) on CodePen.
var dragTarget = document.getElementById('dragTarget');
var mouseup = Rx.Observable.fromEvent(dragTarget, 'mouseup');
var mousemove = Rx.Observable.fromEvent(document, 'mousemove');
var mousedown = Rx.Observable.fromEvent(dragTarget, 'mousedown');
var mousedrag = mousedown.flatMap(function (md) {
var startX = md.offsetX, startY = md.offsetY;
return mousemove.map(function (mm) {
mm.preventDefault();
return {
left: mm.clientX - startX,
top: mm.clientY - startY
};
}).takeUntil(mouseup);
});
var subscription = mousedrag.subscribe(function (pos) {
dragTarget.style.top = pos.top + 'px';
dragTarget.style.left = pos.left + 'px';
});
See the Pen Event Listener by Brian Leathem (@bleathem) on CodePen.
{...a...b...c......d..e.....f...}
{1...2...3......4..5.....6}
|
|
takeUntil
method the mousemove
Observable?{...1.....2....3..4...5....}.take(3)
yields:
{1.....2....3}
take
and takeUntil
methods then let us terminate Observables, allowing us to act on a well-defined set of valuesRx.Observable.fromEvent($input, 'keyup')
.map(function (e) {
return e.target.value; // Project the text from the input
})
.filter(function (text) {
return text.length > 2; // Only if the text is longer than 2 characters
})
.debounce(750 /* Pause for 750ms */ )
.distinctUntilChanged() // Only if the value has changed
.flatMapLatest(searchWikipedia)
.subscribe(function (data) {
// ...
});
See the Pen Event Listener by Brian Leathem (@bleathem) on CodePen.
Quite simply:
Rx.Js allows us to complex asynchronous applications as a composition of very simple functions